Software Environment
Visual Studio Code Installation with WebServer
We will be using the Javascript programming language in our class (and HTML and GLSL within that). The purpose of the class is not to become an expert in Javascript, but rather to use it in the service of practicing coding implementations and debugging in general. To make this as easy as possible, we will start with an Integrated Development Environment (IDE) known as Microsoft Visual Studio Code (VSCode), which will make it easy to organize your projects and to run the code you write. Please visit this link to download VSCode for your appropriate operating system. Most Windows and Linux users will want the 64-bit download. Please post on Discord if you are having any issues installing VSCode.
NOTE: You may also use another editor, but you will have to manually setup a web server locally to avoid cross-origin exceptions for most of the assignments. We did this last year, and it was a pain sometimes.
Live Server Plugin (Strongly Recommended)
VSCode has a ton of awesome, open source plugins. One that's particularly useful for our course is a local web server that can launch your pages in the browser and automatically refresh them as you save your file. Once you have downloaded VSCode, you will need to click on the Extensions
button (circled in red in the image below) and search for Live Server
, and then click install
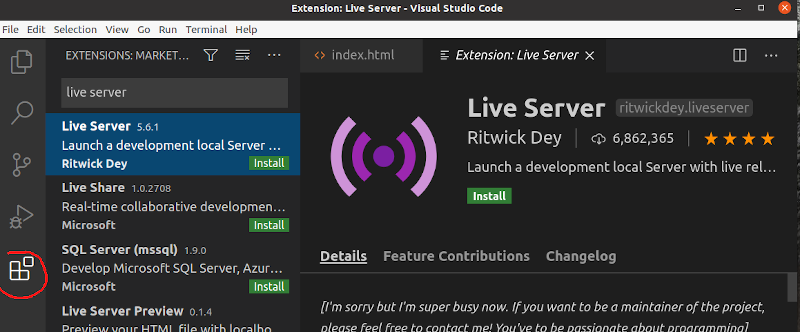
Then, open a top level folder containing the code that you want to run, right click on an HTML file you want to run, and click Open with Live Server
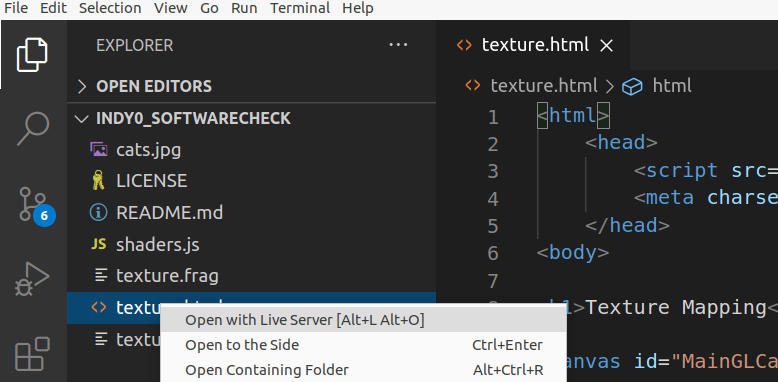
This will then launch your code in a browser window, and as you type and save any code that the HTML page depends on, it will automatically refresh in the browser(!!).
If you want to change the default browser that opens with the live server, then you have to change the settings.json
to have the CustomBrowser
field. For example, my settings.json
file has the following code to get it to launch Chrome by default
The GIF below shows how to access the settings.json
file
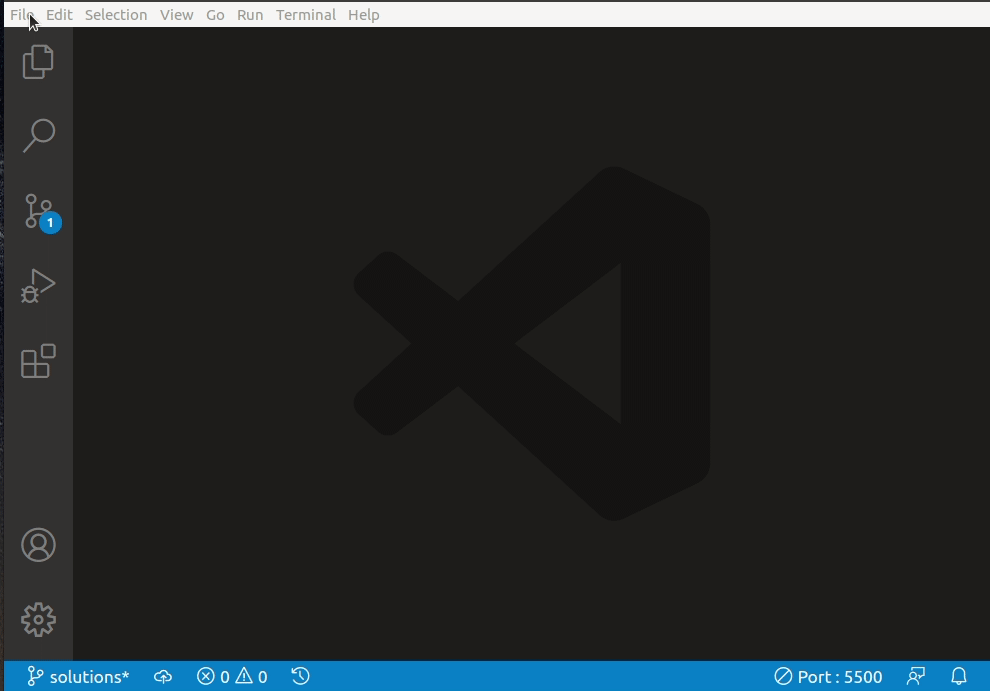
NOTE: The assignments have been tested in Firefox and Chrome, so it's best if you stick to one of those browsers.
Quick Features Overview
Below are a few features I want to highlight that will come in handy as your working.
Unused variable highlighting
If you don't use a variable, it will show up in darker text. This is very useful when debugging. It means that either you didn't actually need the variable, you forgot to use it (hence you didn't complete your code), or you spelled it wrong somewhere. This saved many a student last year!
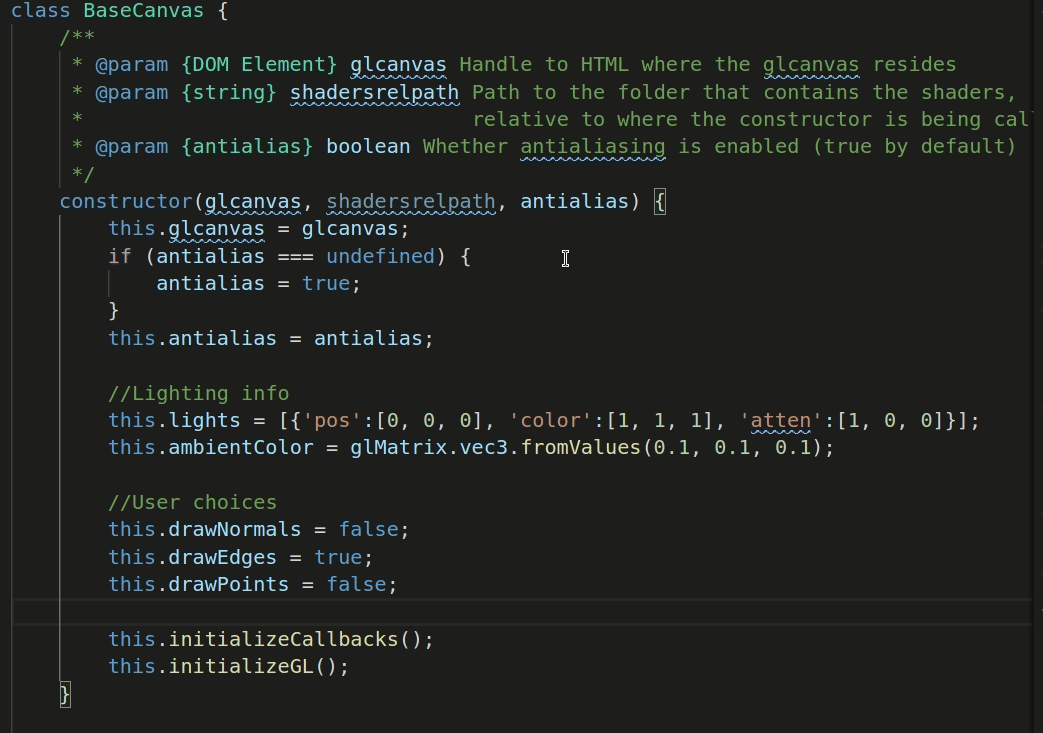
Replace within Selection
If you want to change the name of a variable in one section of your code, you can do a CTRL+F
, which will bring up the find/replace menu, then follow these steps:
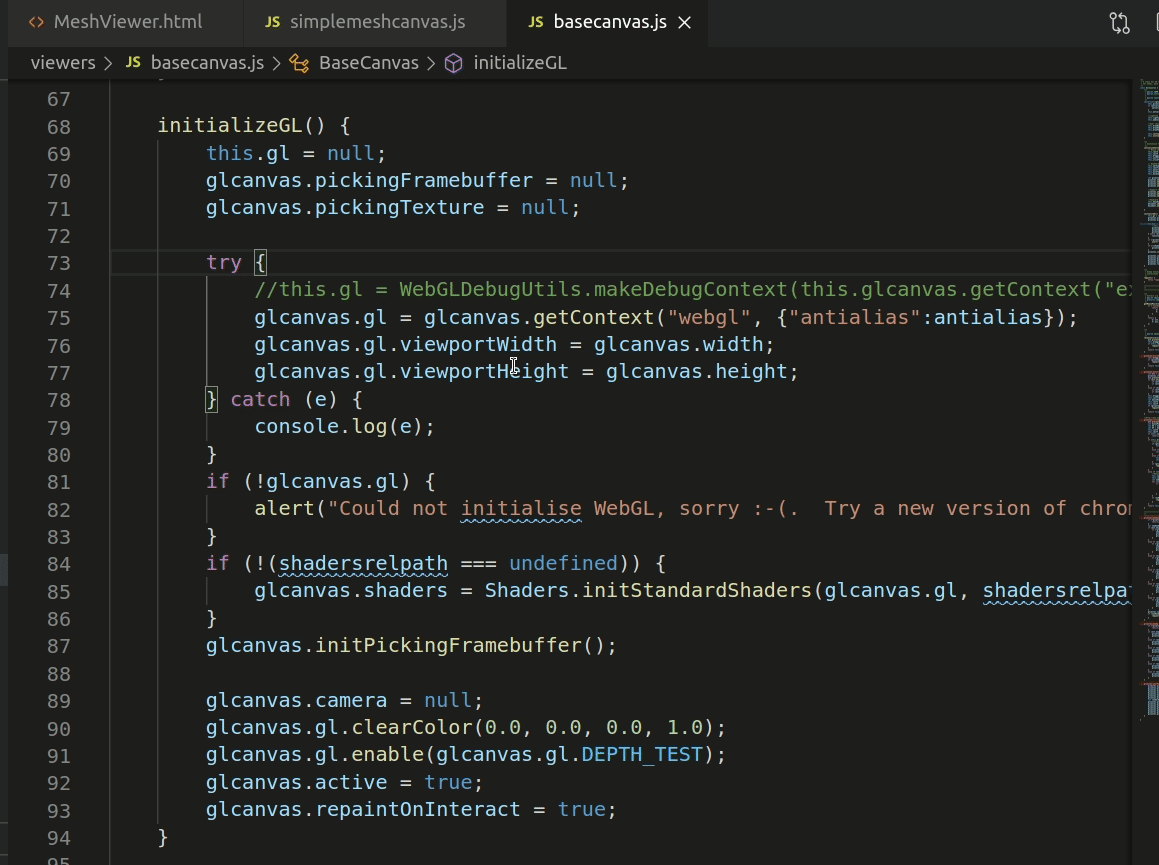
Debugging Javascript
Firefox and Chrome have robust tools built in that you can use to debug your code. On most computers, you can hit F12
to bring up the debugging console. There, you will see any output from console.log()
, and you will be able to set breakpoints in an interactive debugger.
Local web server (Optional)
If you want to run your code without using the built in webserver from VisualStudio cod,e the easiest way to do that is to use Python's built in web server. Download and install Python on your machine if you don't already have it. If you are installing Python for the first time, it is recommended that you use Python 3.7 from Anaconda. Once you have Python installed, open up your console and navigate to the root of the directory that has all of your HTML files. Then type
python -m http.server
python -m SimpleHTTPServer
By default, this will provide access to your code on port 8000 on your local machine, so you would go to the link http://127.0.0.1:8000/ to run your code. Please post on Discord if you are having trouble with this. This was our default way of running code last year, and it was a bit of a pain, particularly because students often had to do a "hard refresh" in their browser to run the most updated code.